Retreat
Before you can go through this example you need to first setup your environment. You can find out how to do that in our Getting started guide .
Sometimes it is good for our units to retreat back to safety, to regroup or regenerate health.
In this example we will create a basic strategy, where if our worker unit sees an opponent warrior unit, it starts driving back to spawn backwards. This is not a very effective strategy and it only demonstrates the concept.
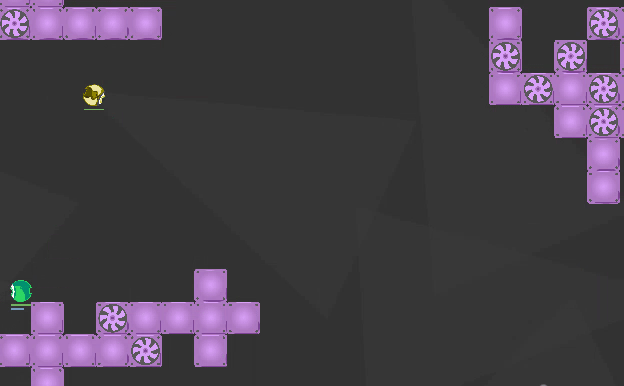
Code
Below is the code for this example.
⚠️ To keep the example clean we didn’t include the whole code for the bot. In order to use this code you need to paste it to an appropriate location in your bot implementation.
|
|
|
|
|
|
Explanation
We iterate through all of our worker units, check through all of their opponents if any of them is a warrior and in case it is, we drive back to spawn backwards.
Note that if you still use the logic of our basic bot and your worker unit is going back to the spawn, has no opponent warrior unit in view and sees a resource, it will stop going to the spawn and go after the resource instead.
♖ Extra tips
Only retreat when low on health - If your unit has full health, don’t make it retreat if it sees an opponent and instead send it to collect resources.
Choose when to drive backwards and forwards - Find a safe location and drive backwards or forwards depending on which way you will reach your destination faster.
Find a better retreat location - You don’t need to retreat way back to the spawn, find a better location instead that may be next to multiple of your units.
Next up
If you want to analyse the map when the game starts, load some external files or something similar it may take some time. We have provided a way where you have more time to setup your bot with everything it needs before the game starts. Check the next example to see how it is done.
Next: Preprate your bot on start